Recipes with Angular.js #
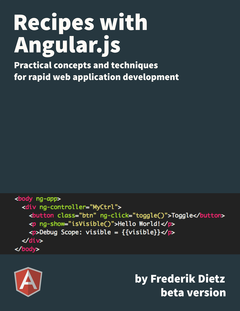
It is free! #
Download the whole course for free.
What you'll learn #
Angular.js is an open-source Javascript MVC (Model-View-Controller) framework developed by Google. It gives Javascript developers a highly structured approach to developing rich browser-based applications which leads to very high productivity.
If you are using Angular.js, or considering it, this cookbook provides easy to follow recipes for issues you are likely to face. Each recipe solves a specific problem and provides a solution and in-depth discussion of it.
Table of Contents #
- Preface
- Introduction
- Code Examples
- How to contact me
- Acknowledgements
- An Introduction to Angular.js
- Including the Angular.js Library Code in an HTML Page
- Binding a Text Input to an Expression
- Responding to Click Events using Controllers
- Converting Expression Output with Filters
- Creating Custom HTML Elements with Directives
- Controllers
- Assigning a Default Value to a Model
- Changing a Model Value with a Controller Function
- Encapsulating a Model Value with a Controller Function
- Responding to Scope Changes
- Sharing Models Between Nested Controllers
- Sharing Code Between Controllers using Services
- Testing Controllers
- Directives
- Enabling/Disabling DOM Elements Conditionally
- Changing the DOM in Response to User Actions
- Rendering an HTML Snippet in a Directive
- Rendering a Directive’s DOM Node Children
- Passing Configuration Params Using HTML Attributes
- Repeatedly Rendering Directive’s DOM Node Children
- Directive-to-Directive Communication
- Testing Directives
- Filters
- Formatting a String With a Currency Filter
- Implementing a Custom Filter to Reverse an Input String
- Passing Configuration Params to Filters
- Filtering a List of DOM Nodes
- Chaining Filters together
- Testing Filters
- Consuming Externals Services
- Requesting JSON Data with AJAX
- Consuming RESTful APIs
- Consuming JSONP APIs
- Deferred and Promise
- Testing Services
- URLs, Routing and Partials
- Client-Side Routing with Hashbang URLs
- Using Regular URLs with the HTML5 History API
- Using Route Location to Implement a Navigation Menu
- Listening on Route Changes to Implement a Login Mechanism
- Using Forms
- Implementing a Basic Form
- Validating a Form Model Client-Side
- Displaying Form Validation Errors
- Displaying Form Validation Errors with the Twitter Bootstrap framework
- Only Enabling the Submit Button if the Form is Valid
- Implementing Custom Validations
- Common User Interface Patterns
- Filtering and Sorting a List
- Paginating Through Client-Side Data
- Paginating Through Server-Side Data
- Paginating Using Infinite Results
- Displaying a Flash Notice/Failure Message
- Editing Text In-Place using HTML5 ContentEditable
- Displaying a Modal Dialog
- Displaying a Loading Spinner
- Backend Integration with Ruby on Rails
- Consuming REST APIs
- Implementing Client-Side Routing
- Validating Forms Server-Side
- Backend Integration with Node Express
- Consuming REST APIs
- Implementing Client-Side Routing